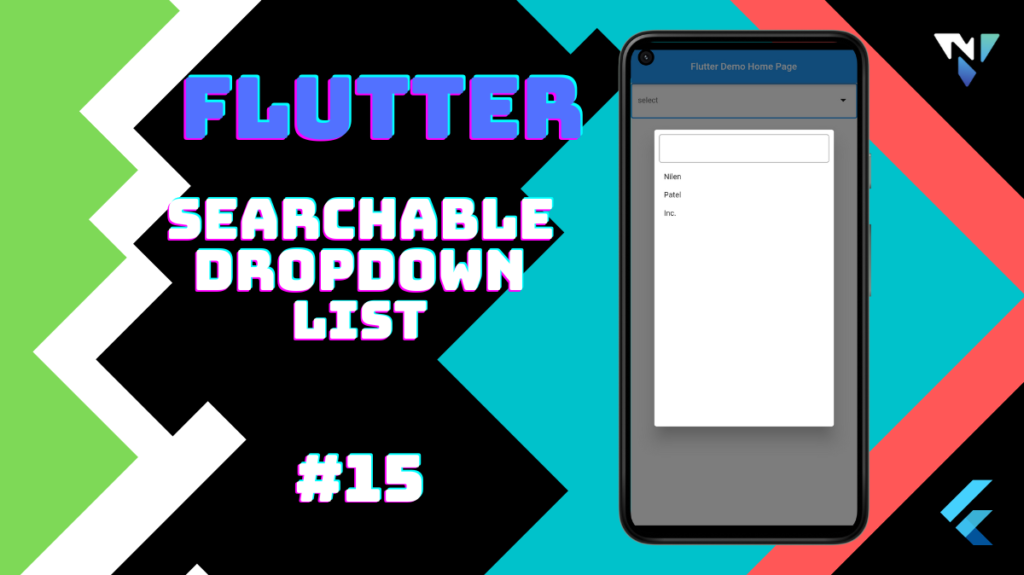
Tutorial and code of searchable dropdown list in flutter. Copy and paste the below code as per your requirements.
dropdown_search: ^0.4.8
import 'package:dropdown_search/dropdown_search.dart';
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key? key, required this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
late KeyValueModel selectedValue;
late String hello;
TextEditingController v = TextEditingController();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Center(child: Text(widget.title),),
),
body: SingleChildScrollView(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
DropdownSearch<KeyValueModel>(
mode: Mode.DIALOG,
showSelectedItem:false,
items: [KeyValueModel(itemId: "01",itemName: "Nilen"),KeyValueModel(itemId: "02",itemName: "Patel"),KeyValueModel(itemId: "02",itemName: "Inc.")],
itemAsString: (KeyValueModel u) => u.itemName,
hint: "select",
onChanged: (value){
v.text = value.itemId.toString();
print(value.itemId);
},
showSearchBox: true,
filterFn: (instance, filter){
if(instance.itemName.contains(filter)){
print(filter);
return true;
}
else{
return false;
}
},
popupItemBuilder: (context,KeyValueModel item,bool isSelected){
return Container(
margin: EdgeInsets.symmetric(horizontal: 8),
decoration: !isSelected
? null
: BoxDecoration(
border: Border.all(color: Theme.of(context).primaryColor),
borderRadius: BorderRadius.circular(5),
color: Colors.white,
),
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Text(item.itemName),
),
);
},
),
],
),
),
),
);
}
}
class KeyValueModel{
String itemName;
String itemId;
KeyValueModel({required this.itemName,required this.itemId});
}
Leave a Reply