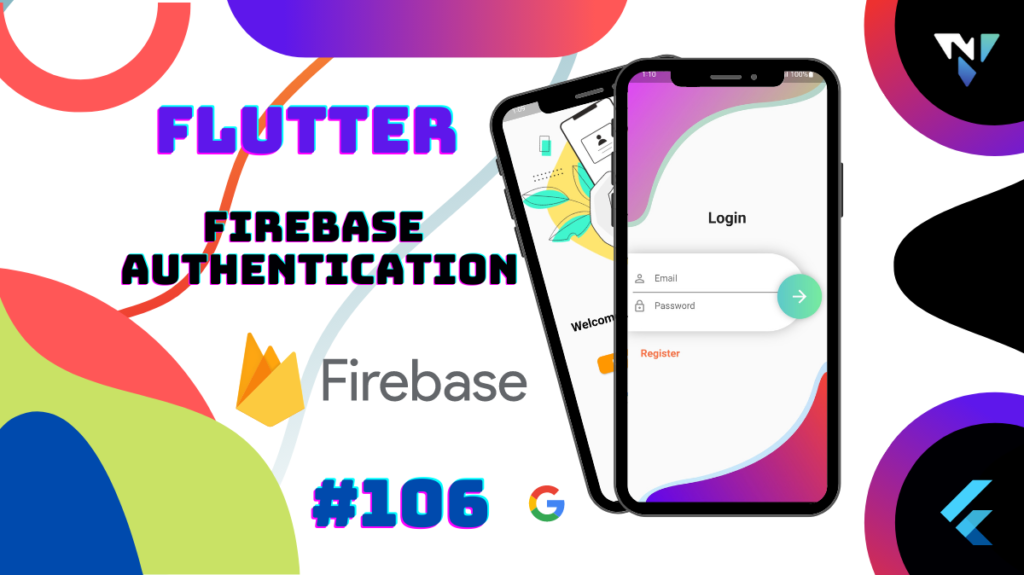
Tutorial of Firebase Authentication from Scratch. Copy and paste the below code as per your requirements.
pubspec.yaml
firebase_auth:
flutter_signin_button:
firebase_core:
google_sign_in:
components/curved-left.dart
import 'package:flutter/material.dart';
class CurvedLeft extends StatelessWidget {
@override
Widget build(BuildContext context) {
Size size = MediaQuery.of(context).size;
return Container(
child: ClipPath(
clipper: LeftClipper(),
child: Container(
height: 300.0,
width: size.width,
decoration: const BoxDecoration(
gradient: LinearGradient(
begin: Alignment.topLeft,
end: Alignment.bottomRight,
colors: [
Colors.purpleAccent,
Colors.lightGreenAccent
],
),
),
),
),
);
}
}
class LeftClipper extends CustomClipper<Path> {
@override
Path getClip(Size size) {
Path path = Path();
path.lineTo(0, 0);
path.lineTo(0, size.height);
path.quadraticBezierTo(30, size.height, 40, size.height - 40);
path.quadraticBezierTo(80, 80, size.width - 120, 70);
path.quadraticBezierTo(size.width, 60, size.width, 0);
path.lineTo(size.width, 0);
return path;
}
@override
bool shouldReclip(CustomClipper<Path> oldClipper) {
return false;
}
}
components/curved-left-shadow.dart
import 'package:flutter/material.dart';
class CurvedLeftShadow extends StatelessWidget {
const CurvedLeftShadow({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
Size size = MediaQuery.of(context).size;
return ClipPath(
clipper: LeftShadowClipper(),
child: Container(
height: 320.0,
width: size.width,
decoration: const BoxDecoration(
gradient: LinearGradient(
begin: Alignment.topLeft,
end: Alignment.bottomRight,
colors: [
Color.fromRGBO(43, 169, 95, 0.3),
Color.fromRGBO(5, 101, 91, 0.3)
],
),
),
),
);
}
}
class LeftShadowClipper extends CustomClipper<Path> {
@override
Path getClip(Size size) {
Path path = Path();
path.lineTo(0, 0);
path.lineTo(0, size.height - 10);
path.quadraticBezierTo(35, size.height - 10, 45, size.height - 50);
path.quadraticBezierTo(90, 90, size.width - 120, 80);
path.quadraticBezierTo(size.width, 70, size.width, 25);
path.lineTo(size.width, 0);
return path;
}
@override
bool shouldReclip(CustomClipper<Path> oldClipper) {
return false;
}
}
components/curved-right.dart
import 'package:flutter/material.dart';
class CurvedRight extends StatelessWidget {
const CurvedRight({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
Size size = MediaQuery.of(context).size;
return ClipPath(
clipper: RightClipper(),
child: Container(
height: 250.0,
width: size.width,
decoration: const BoxDecoration(
gradient: LinearGradient(
begin: Alignment.bottomLeft,
end: Alignment.topRight,
colors: [
Color.fromRGBO(86, 1, 251, 1.0),
Colors.red
],
),
),
),
);
}
}
class RightClipper extends CustomClipper<Path> {
@override
Path getClip(Size size) {
Path path = Path();
path.lineTo(35, size.height);
path.quadraticBezierTo(
40,
size.height - 25,
110,
size.height - 35,
);
path.quadraticBezierTo(
size.width - 60,
size.height - 70,
size.width - 19,
35,
);
path.quadraticBezierTo(
size.width - 10,
0,
size.width,
0,
);
path.lineTo(size.width, size.height);
path.lineTo(35, size.height);
return path;
}
@override
bool shouldReclip(CustomClipper<Path> oldClipper) {
return false;
}
}
components/curved-right-shadow.dart
import 'package:flutter/material.dart';
class CurvedRightShadow extends StatelessWidget {
@override
Widget build(BuildContext context) {
Size size = MediaQuery.of(context).size;
return Container(
child: ClipPath(
clipper: RightShadowClipper(),
child: Container(
height: 270.0,
width: size.width,
decoration: BoxDecoration(
gradient: LinearGradient(
begin: Alignment.bottomLeft,
end: Alignment.topRight,
colors: [
Color.fromRGBO(86, 201, 251, 0.3),
Color.fromRGBO(76, 170, 251, 0.3)
],
),
),
),
),
);
}
}
class RightShadowClipper extends CustomClipper<Path> {
@override
Path getClip(Size size) {
Path path = Path();
path.lineTo(20, size.height);
path.quadraticBezierTo(
40,
size.height - 35,
130,
size.height - 47,
);
path.quadraticBezierTo(
size.width - 60,
size.height - 90,
size.width - 25,
40,
);
path.quadraticBezierTo(
size.width - 10,
0,
size.width,
0,
);
path.lineTo(size.width, size.height);
path.lineTo(20, size.height);
return path;
}
@override
bool shouldReclip(CustomClipper<Path> oldClipper) {
return true;
}
}
start.dart
import 'package:firebase_auth/firebase_auth.dart';
import 'package:flutter/material.dart';
import 'package:flutter_signin_button/flutter_signin_button.dart';
import 'package:google_sign_in/google_sign_in.dart';
class Start extends StatefulWidget {
const Start({Key? key}) : super(key: key);
@override
_StartState createState() => _StartState();
}
class _StartState extends State<Start> {
final FirebaseAuth _auth = FirebaseAuth.instance;
Future<UserCredential> googleSignIn() async {
GoogleSignIn googleSignIn = GoogleSignIn();
GoogleSignInAccount? googleUser = await googleSignIn.signIn();
if (googleUser != null) {
GoogleSignInAuthentication googleAuth = await googleUser.authentication;
if (googleAuth.idToken != null && googleAuth.accessToken != null) {
final AuthCredential credential = GoogleAuthProvider.credential(
accessToken: googleAuth.accessToken, idToken: googleAuth.idToken);
final UserCredential user =
await _auth.signInWithCredential(credential);
await Navigator.pushReplacementNamed(context, "/");
return user;
} else {
throw StateError('Missing Google Auth Token');
}
} else {
throw StateError('Sign in Aborted');
}
}
navigateToLogin() async {
Navigator.pushReplacementNamed(context, "Login");
}
navigateToRegister() async {
Navigator.pushReplacementNamed(context, "SignUp");
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Column(
children: <Widget>[
const SizedBox(height: 35.0),
const SizedBox(
height: 400,
child: Image(
image: AssetImage("assets/illustration.png"),
fit: BoxFit.contain,
),
),
const SizedBox(height: 20),
RichText(
text: const TextSpan(
text: 'Welcome to ',
style: TextStyle(
fontSize: 25.0,
fontWeight: FontWeight.bold,
color: Colors.black),
children: <TextSpan>[
TextSpan(
text: 'Firebase Auth',
style: TextStyle(
fontSize: 30.0,
fontWeight: FontWeight.bold,
color: Colors.orange))
])),
const SizedBox(height: 50.0),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
RaisedButton(
padding: const EdgeInsets.only(left: 30, right: 30),
onPressed: navigateToLogin,
child: const Text(
'LOGIN',
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
color: Colors.white,
),
),
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10.0),
),
color: Colors.orange),
const SizedBox(width: 20.0),
RaisedButton(
padding: const EdgeInsets.only(left: 30, right: 30),
onPressed: navigateToRegister,
child: const Text(
'REGISTER',
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
color: Colors.white,
),
),
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10.0),
),
color: Colors.orange),
],
),
const SizedBox(height: 20.0),
SignInButton(Buttons.Google,
text: "Sign up with Google", onPressed: googleSignIn)
],
),
);
}
}
HomePage.dart
import 'package:example/Start.dart';
import 'package:firebase_auth/firebase_auth.dart';
import 'package:flutter/material.dart';
// import 'package:authentification/Start.dart';
import 'package:google_sign_in/google_sign_in.dart';
class HomePage extends StatefulWidget {
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
final FirebaseAuth _auth = FirebaseAuth.instance;
late User user;
bool isloggedin = false;
checkAuthentification() async {
_auth.authStateChanges().listen((user) {
if (user == null) {
Navigator.of(context).pushReplacementNamed("start");
}
});
}
getUser() async {
User? firebaseUser = _auth.currentUser;
await firebaseUser?.reload();
firebaseUser = _auth.currentUser;
if (firebaseUser != null) {
setState(() {
this.user = firebaseUser!;
this.isloggedin = true;
});
}
}
signOut() async {
_auth.signOut();
final googleSignIn = GoogleSignIn();
await googleSignIn.signOut();
}
@override
void initState() {
super.initState();
this.checkAuthentification();
this.getUser();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Container(
child: !isloggedin
? CircularProgressIndicator()
: Column(
children: <Widget>[
SizedBox(height: 40.0),
Container(
height: 300,
child: Image(
image: AssetImage("assets/background.png"),
fit: BoxFit.contain,
),
),
Container(
child: Text(
"Hello ${user.displayName} you are Logged in as ${user.email}",
style:
TextStyle(fontSize: 20.0, fontWeight: FontWeight.bold),
),
),
RaisedButton(
padding: EdgeInsets.fromLTRB(70, 10, 70, 10),
onPressed: signOut,
child: Text('Signout',
style: TextStyle(
color: Colors.white,
fontSize: 20.0,
fontWeight: FontWeight.bold)),
color: Colors.orange,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20.0),
),
)
],
),
));
}
}
SignUp.dart
import 'package:flutter/material.dart';
import 'package:example/components/curved-left-shadow.dart';
import 'package:example/components/curved-left.dart';
import 'package:example/components/curved-right-shadow.dart';
import 'package:example/components/curved-right.dart';
import 'package:firebase_auth/firebase_auth.dart';
class SignUp extends StatefulWidget {
const SignUp({Key? key}) : super(key: key);
@override
_SignUpState createState() => _SignUpState();
}
class _SignUpState extends State<SignUp> {
final FirebaseAuth _auth = FirebaseAuth.instance;
final GlobalKey<FormState> _formKey = GlobalKey<FormState>();
late String _name, _email, _password;
checkAuthentication() async {
_auth.authStateChanges().listen((user) async {
if (user != null) {
Navigator.pushReplacementNamed(context, "/");
}
});
}
@override
void initState() {
super.initState();
checkAuthentication();
}
signUp() async {
if (_formKey.currentState!.validate()) {
_formKey.currentState!.save();
try {
UserCredential user = await _auth.createUserWithEmailAndPassword(
email: _email, password: _password);
if (user != null) {
await _auth.currentUser!.updateProfile(displayName: _name);
}
} catch (e) {
showError(e.toString());
print(e);
}
}
}
showError(String errormessage) {
showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: const Text('ERROR'),
content: Text(errormessage),
actions: <Widget>[
ElevatedButton(
onPressed: () {
Navigator.of(context).pop();
},
child: const Text('OK'))
],
);
});
}
@override
Widget build(BuildContext context) {
Size size = MediaQuery.of(context).size;
return Scaffold(
body: SingleChildScrollView(
child: Form(
key: _formKey,
child: Stack(
children: <Widget>[
Positioned(top: 0, left: 0, child: CurvedLeftShadow()),
Positioned(top: 0, left: 0, child: CurvedLeft()),
Positioned(bottom: 0, left: 0, child: CurvedRightShadow()),
Positioned(bottom: 0, left: 0, child: CurvedRight()),
SizedBox(
height: size.height,
width: size.width,
child: Column(
mainAxisAlignment: MainAxisAlignment.start,
children: <Widget>[
GestureDetector(
onTap: () {
Navigator.pushNamed(context, "Login");
},
child: Container(
alignment: Alignment.centerRight,
padding: const EdgeInsets.only(
right: 30.0,
top: 160.0,
bottom: 50.0
),
child: const Text(
"Login",
style: TextStyle(
fontSize: 25.0,
color: Colors.deepOrangeAccent,
fontWeight: FontWeight.w700,
),
),
),
),
const Padding(
padding: EdgeInsets.only(bottom: 55.0),
child: Text(
"Register",
style: TextStyle(fontSize: 37.0, fontWeight: FontWeight.w700),
),
),
Stack(
children: <Widget>[
Container(
height: 180.0,
padding: const EdgeInsets.only(left: 10.0),
margin: const EdgeInsets.only(right: 40.0),
decoration: const BoxDecoration(
color: Colors.white,
boxShadow: [
BoxShadow(
color: Colors.black26,
blurRadius: 20.0,
)
],
borderRadius: BorderRadius.only(
topRight: Radius.circular(90.0),
bottomRight: Radius.circular(90.0),
),
),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
TextFormField(
validator: (input) {
if (input!.isEmpty) return 'Enter Name';
},
style: const TextStyle(fontSize: 22.0),
decoration: const InputDecoration(
contentPadding: EdgeInsets.symmetric(
vertical: 15.0,
),
icon: Icon(
Icons.person_outline,
size: 26.0,
),
hintText: "Name",
border: InputBorder.none,
),
onSaved: (input) => _name = input!
),
Container(
decoration: BoxDecoration(
border: Border.all(
color: Colors.grey,
),
),
),
TextFormField(
validator: (input) {
if (input!.isEmpty) return 'Enter Email';
},
style: const TextStyle(fontSize: 22.0),
decoration: const InputDecoration(
contentPadding: EdgeInsets.symmetric(
vertical: 15.0,
),
icon: Icon(
Icons.email,
size: 26.0,
),
hintText: "Email",
border: InputBorder.none,
),
onSaved: (input) => _email = input!
),
Container(
decoration: BoxDecoration(
border: Border.all(
color: Colors.grey,
),
),
),
TextFormField(
validator: (input) {
if (input!.length < 6) {
return 'Provide Minimum 6 Character';
}
},
style: const TextStyle(fontSize: 22.0),
decoration: const InputDecoration(
contentPadding: EdgeInsets.symmetric(
vertical: 15.0,
),
icon: Icon(
Icons.lock_outlined,
size: 26.0,
),
hintText: "Password",
border: InputBorder.none,
),
obscureText: true,
onSaved: (input) => _password = input!
),
],
),
),
Positioned(
top: 55,
right: 10,
child: GestureDetector(
onTap: signUp,
child: Container(
padding: const EdgeInsets.all(15.0),
decoration: const BoxDecoration(
shape: BoxShape.circle,
gradient: LinearGradient(
begin: Alignment.centerLeft,
end: Alignment.centerRight,
colors: [
Color.fromRGBO(94, 201, 202, 1.0),
Color.fromRGBO(119, 235, 159, 1.0),
],
),
boxShadow: [
BoxShadow(
color: Colors.black26,
blurRadius: 10.0,
)
],
),
child: const Icon(
Icons.check,
size: 40.0,
color: Colors.white,
),
),
)
),
],
),
],
),
),
],
),
),
)
);
}
}
Login.dart
import 'package:flutter/material.dart';
import 'package:firebase_auth/firebase_auth.dart';
import 'SignUp.dart';
import 'components/curved-left-shadow.dart';
import 'components/curved-left.dart';
import 'components/curved-right-shadow.dart';
import 'components/curved-right.dart';
class Login extends StatefulWidget {
@override
_LoginState createState() => _LoginState();
}
class _LoginState extends State<Login> {
final FirebaseAuth _auth = FirebaseAuth.instance;
final GlobalKey<FormState> _formKey = GlobalKey<FormState>();
late String _email, _password;
checkAuthentification() async {
_auth.authStateChanges().listen((user) {
if (user != null) {
print(user);
Navigator.pushReplacementNamed(context, "/");
}
});
}
@override
void initState() {
super.initState();
this.checkAuthentification();
}
login() async {
if (_formKey.currentState!.validate()) {
_formKey.currentState!.save();
try {
await _auth.signInWithEmailAndPassword(
email: _email, password: _password);
} catch (e) {
showError(e.toString());
print(e);
}
}
}
showError(String errormessage) {
showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: const Text('ERROR'),
content: Text(errormessage),
actions: <Widget>[
FlatButton(
onPressed: () {
Navigator.of(context).pop();
},
child: const Text('OK'))
],
);
});
}
navigateToSignUp() async {
Navigator.push(context, MaterialPageRoute(builder: (context) => SignUp()));
}
@override
@override
Widget build(BuildContext context) {
Size size = MediaQuery.of(context).size;
return Scaffold(
body: Container(
child: Stack(
children: <Widget>[
Positioned(top: 0, left: 0, child: CurvedLeftShadow()),
Positioned(top: 0, left: 0, child: CurvedLeft()),
Positioned(bottom: 0, left: 0, child: CurvedRightShadow()),
Positioned(bottom: 0, left: 0, child: CurvedRight()),
Form(
key: _formKey,
child: Container(
height: size.height,
width: size.width,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Padding(
padding: EdgeInsets.only(bottom: 50.0),
child: Text(
"Login",
style: TextStyle(fontSize: 37.0, fontWeight: FontWeight.w700),
),
),
Stack(
children: <Widget>[
Container(
height: 150.0,
padding: const EdgeInsets.only(left: 10.0),
margin: const EdgeInsets.only(right: 40.0),
decoration: const BoxDecoration(
color: Colors.white,
boxShadow: [
BoxShadow(
color: Colors.black26,
blurRadius: 20.0,
)
],
borderRadius: BorderRadius.only(
topRight: Radius.circular(90.0),
bottomRight: Radius.circular(90.0),
),
),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
TextFormField(
validator: (input) {
if (input!.isEmpty) return 'Enter Email';
},
style: const TextStyle(fontSize: 22.0),
decoration: const InputDecoration(
contentPadding: EdgeInsets.symmetric(
vertical: 15.0,
),
icon: Icon(
Icons.person_outline,
size: 26.0,
),
hintText: "Email",
border: InputBorder.none,
),
onSaved: (input) => _email = input!,
),
Container(
decoration: BoxDecoration(
border: Border.all(
color: Colors.grey,
),
),
),
TextFormField(
validator: (input) {
if (input!.length < 6)
return 'Provide Minimum 6 Character';
},
style: const TextStyle(fontSize: 22.0),
decoration: const InputDecoration(
contentPadding: EdgeInsets.symmetric(
vertical: 15.0,
),
icon: Icon(
Icons.lock_outline,
size: 26.0,
),
hintText: "Password",
border: InputBorder.none,
),
obscureText: true,
onSaved: (input) => _password = input!,
),
],
),
),
Positioned(
top: 40,
right: 10,
child: Container(
padding: const EdgeInsets.all(15.0),
decoration: const BoxDecoration(
shape: BoxShape.circle,
gradient: LinearGradient(
begin: Alignment.centerLeft,
end: Alignment.centerRight,
colors: [
Color.fromRGBO(94, 201, 202, 1.0),
Color.fromRGBO(119, 235, 159, 1.0),
],
),
boxShadow: [
BoxShadow(
color: Colors.black26,
blurRadius: 10.0,
)
],
),
child: IconButton(
icon:const Icon(Icons.arrow_forward),
iconSize: 40.0,
color: Colors.white,
onPressed: login,
),
),
),
],
),
Container(
alignment: Alignment.centerLeft,
padding: const EdgeInsets.only(
left: 25.0,
top: 30.0,
),
child: GestureDetector(
onTap: () {
Navigator.pushNamed(context, "SignUp");
},
child: const Text(
"Register",
style: TextStyle(
fontSize: 25.0,
color: Colors.deepOrangeAccent,
fontWeight: FontWeight.w700,
),
),
),
),
],
),
),
)
],
),
),
);
}
}
main.dart
import 'package:example/Login.dart';
import 'package:example/SignUp.dart';
import 'package:example/Start.dart';
import 'package:flutter/material.dart';
import 'HomePage.dart';
import 'package:firebase_core/firebase_core.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(
primaryColor: Colors.orange
),
debugShowCheckedModeBanner: false,
home:
HomePage(),
routes: <String,WidgetBuilder>{
"Login" : (BuildContext context)=>Login(),
"SignUp":(BuildContext context)=>const SignUp(),
"start":(BuildContext context)=>const Start(),
},
);
}
}