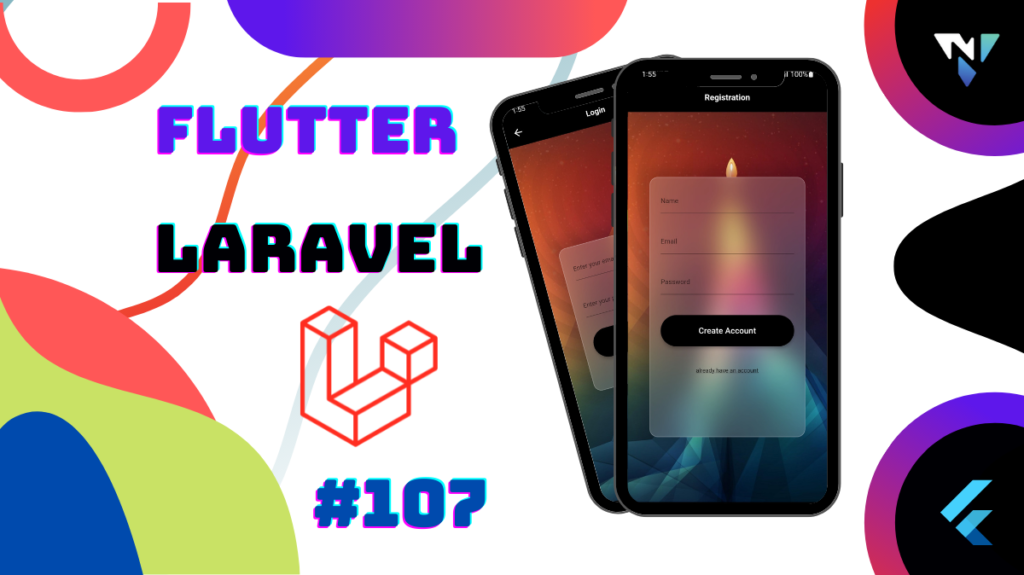
Flutter Laravel Backend PHP | Flutter Rest API | Flutter Http Post Request. Copy and paste the below code as per your requirements.
api.php
Route::post('/auth/register', [AuthController::class, 'register']);
Route::post('/auth/login', [AuthController::class, 'login']);
AuthController.php
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Hash;
use Illuminate\Support\Facades\Validator;
class AuthController extends Controller
{
public function register(Request $req)
{
//valdiate
$rules = [
'name' => 'required|string',
'email' => 'required|string|unique:users',
'password' => 'required|string|min:6'
];
$validator = Validator::make($req->all(), $rules);
if ($validator->fails()) {
return response()->json($validator->errors(), 400);
}
//create new user in users table
$user = User::create([
'name' => $req->name,
'email' => $req->email,
'password' => Hash::make($req->password)
]);
$token = $user->createToken('Personal Access Token')->plainTextToken;
$response = ['user' => $user, 'token' => $token];
return response()->json($response, 200);
}
public function login(Request $req)
{
// validate inputs
$rules = [
'email' => 'required',
'password' => 'required|string'
];
$req->validate($rules);
// find user email in users table
$user = User::where('email', $req->email)->first();
// if user email found and password is correct
if ($user && Hash::check($req->password, $user->password)) {
$token = $user->createToken('Personal Access Token')->plainTextToken;
$response = ['user' => $user, 'token' => $token];
return response()->json($response, 200);
}
$response = ['message' => 'Incorrect email or password'];
return response()->json($response, 400);
}
}
.env.example
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=auth_app
DB_USERNAME=root
DB_PASSWORD=
Commands on Terminal
sudo artisan migrate
sudo php artisan serve --host 192.168.0.104 --port 8000
main.dart
import 'package:flutter/material.dart';
import 'package:example/Screens/register_screen.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
home: RegisterScreen(),
);
}
}
rounded_button.dart
import 'package:flutter/material.dart';
class RoundedButton extends StatelessWidget {
final String btnText;
final Function onBtnPressed;
const RoundedButton(
{Key? key, required this.btnText, required this.onBtnPressed})
: super(key: key);
@override
Widget build(BuildContext context) {
return Material(
elevation: 5,
color: Colors.black,
borderRadius: BorderRadius.circular(30),
child: MaterialButton(
onPressed: () {
onBtnPressed();
},
minWidth: 320,
height: 60,
child: Text(
btnText,
style: const TextStyle(color: Colors.white, fontSize: 20),
),
),
);
}
}
Screens/home_screen.dart
import 'package:flutter/material.dart';
class HomeScreen extends StatelessWidget {
const HomeScreen({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const Scaffold(
backgroundColor: Colors.blue,
body: Center(
child: Text('Home Screen'),
),
);
}
}
Screens/login_screen.dart
import 'dart:convert';
import 'dart:ui';
import 'package:flutter/material.dart';
import 'package:example/Services/auth_services.dart';
import 'package:example/Services/globals.dart';
import 'package:example/rounded_button.dart';
import 'package:http/http.dart' as http;
import 'home_screen.dart';
class LoginScreen extends StatefulWidget {
const LoginScreen({Key? key}) : super(key: key);
@override
_LoginScreenState createState() => _LoginScreenState();
}
class _LoginScreenState extends State<LoginScreen> {
String _email = '';
String _password = '';
loginPressed() async {
if (_email.isNotEmpty && _password.isNotEmpty) {
http.Response response = await AuthServices.login(_email, _password);
Map responseMap = jsonDecode(response.body);
if (response.statusCode == 200) {
Navigator.push(
context,
MaterialPageRoute(
builder: (BuildContext context) => const HomeScreen(),
));
} else {
errorSnackBar(context, responseMap.values.first);
}
} else {
errorSnackBar(context, 'enter all required fields');
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.black,
centerTitle: true,
elevation: 0,
title: const Text(
'Login',
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
),
),
),
body: Container(
decoration: const BoxDecoration(
image: DecorationImage(
image: AssetImage("assets/background.png"),
fit: BoxFit.cover,
),
),
child: Center(
child: Container(
decoration: BoxDecoration(boxShadow: [
BoxShadow(
blurRadius: 24,
spreadRadius: 16,
color: Colors.black.withOpacity(0.2),
)
]),
child: ClipRRect(
borderRadius: BorderRadius.circular(16.0),
child: BackdropFilter(
filter: ImageFilter.blur(
sigmaX: 20.0,
sigmaY: 20.0,
),
child: Container(
height: 300,
width: 300,
decoration: BoxDecoration(
color: Colors.white.withOpacity(0.2),
borderRadius: BorderRadius.circular(16.0),
border: Border.all(
width: 1.5,
color: Colors.white.withOpacity(0.2),
)),
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: 20),
child: Column(
children: [
const SizedBox(
height: 20,
),
TextField(
decoration: const InputDecoration(
hintText: 'Enter your email',
),
onChanged: (value) {
_email = value;
},
),
const SizedBox(
height: 30,
),
TextField(
obscureText: true,
decoration: const InputDecoration(
hintText: 'Enter your password',
),
onChanged: (value) {
_password = value;
},
),
const SizedBox(
height: 30,
),
RoundedButton(
btnText: 'LOG IN',
onBtnPressed: () => loginPressed(),
)
],
),
),
),
),
),
),
),
),
);
}
}
Screens/register_screen.dart
import 'dart:convert';
import 'dart:ui';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:example/Services/auth_services.dart';
import 'package:example/Services/globals.dart';
import '../rounded_button.dart';
import 'home_screen.dart';
import 'login_screen.dart';
import 'package:http/http.dart' as http;
class RegisterScreen extends StatefulWidget {
const RegisterScreen({Key? key}) : super(key: key);
@override
_RegisterScreenState createState() => _RegisterScreenState();
}
class _RegisterScreenState extends State<RegisterScreen> {
String _email = '';
String _password = '';
String _name = '';
createAccountPressed() async {
bool emailValid = RegExp(
r"^[a-zA-Z0-9.a-zA-Z0-9.!#$%&'*+-/=?^_`{|}~]+@[a-zA-Z0-9]+\.[a-zA-Z]+")
.hasMatch(_email);
if (emailValid) {
http.Response response =
await AuthServices.register(_name, _email, _password);
Map responseMap = jsonDecode(response.body);
if (response.statusCode == 200) {
Navigator.push(
context,
MaterialPageRoute(
builder: (BuildContext context) => const HomeScreen(),
));
} else {
errorSnackBar(context, responseMap.values.first[0]);
}
} else {
errorSnackBar(context, 'email not valid');
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.black,
centerTitle: true,
elevation: 0,
title: const Text(
'Registration',
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
),
),
),
body: Container(
decoration: const BoxDecoration(
image: DecorationImage(
image: AssetImage("assets/background.png"),
fit: BoxFit.cover,
),
),
child: Center(
child: Container(
decoration: BoxDecoration(boxShadow: [
BoxShadow(
blurRadius: 24,
spreadRadius: 16,
color: Colors.black.withOpacity(0.2),
)
]),
child: ClipRRect(
borderRadius: BorderRadius.circular(16.0),
child: BackdropFilter(
filter: ImageFilter.blur(
sigmaX: 20.0,
sigmaY: 20.0,
),
child: Container(
height: 500,
width: 300,
decoration: BoxDecoration(
color: Colors.white.withOpacity(0.2),
borderRadius: BorderRadius.circular(16.0),
border: Border.all(
width: 1.5,
color: Colors.white.withOpacity(0.2),
)),
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: 20),
child: Column(
children: [
const SizedBox(
height: 20,
),
TextField(
decoration: const InputDecoration(
hintText: 'Name',
),
onChanged: (value) {
_name = value;
},
),
const SizedBox(
height: 30,
),
TextField(
decoration: const InputDecoration(
hintText: 'Email',
),
onChanged: (value) {
_email = value;
},
),
const SizedBox(
height: 30,
),
TextField(
obscureText: true,
decoration: const InputDecoration(
hintText: 'Password',
),
onChanged: (value) {
_password = value;
},
),
const SizedBox(
height: 40,
),
RoundedButton(
btnText: 'Create Account',
onBtnPressed: () => createAccountPressed(),
),
const SizedBox(
height: 40,
),
GestureDetector(
onTap: () {
Navigator.push(
context,
MaterialPageRoute(
builder: (BuildContext context) => const LoginScreen(),
));
},
child: const Text(
'already have an account',
style: TextStyle(
decoration: TextDecoration.underline,
),
),
)
],
),
),
),
),
),
),
),
),
);
}
}
Services/auth_services.dart
import 'dart:convert';
import 'package:example/Services/globals.dart';
import 'package:http/http.dart' as http;
class AuthServices {
static Future<http.Response> register(
String name, String email, String password) async {
Map data = {
"name": name,
"email": email,
"password": password,
};
var body = json.encode(data);
var url = Uri.parse(baseURL + 'auth/register');
http.Response response = await http.post(
url,
headers: headers,
body: body,
);
print(response.body);
return response;
}
static Future<http.Response> login(String email, String password) async {
Map data = {
"email": email,
"password": password,
};
var body = json.encode(data);
var url = Uri.parse(baseURL + 'auth/login');
http.Response response = await http.post(
url,
headers: headers,
body: body,
);
print(response.body);
return response;
}
}
Services/globals.dart
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
const String baseURL = "http://192.168.0.104:8000/api/"; //emulator localhost
const Map<String, String> headers = {"Content-Type": "application/json"};
errorSnackBar(BuildContext context, String text) {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
backgroundColor: Colors.red,
content: Text(text),
duration: const Duration(seconds: 1),
));
}
Leave a Reply