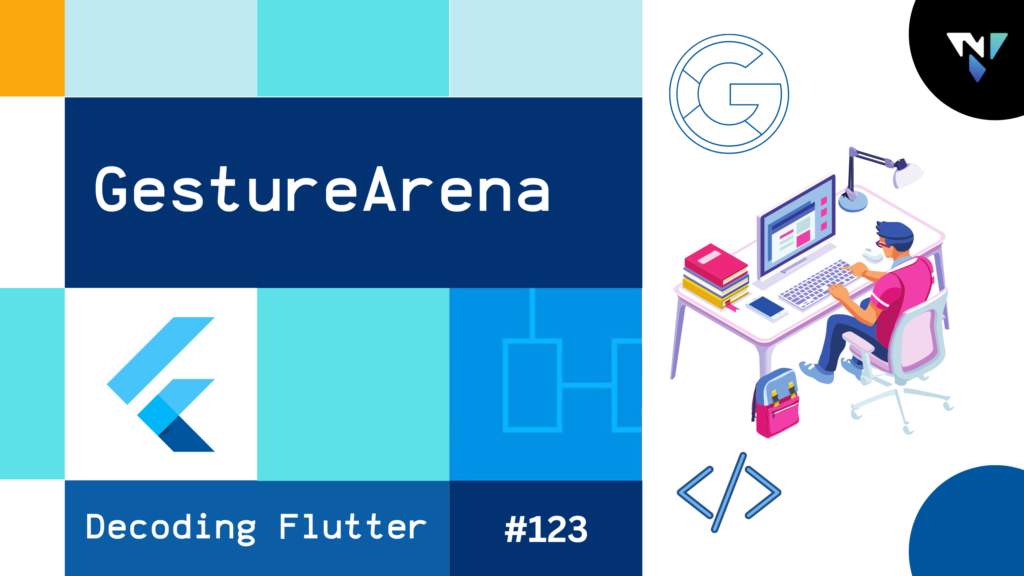
Gesture Arena | Decoding Flutter. Copy and paste the below code as per your requirements.
import 'package:awesome_snackbar_content/awesome_snackbar_content.dart';
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Awesome SnackBar',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(title: 'Awesome SnackBar'),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
// Here we take the value from the MyHomePage object that was created by
// the App.build method, and use it to set our appbar title.
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
ElevatedButton(
onPressed: (){
final snackBar = SnackBar(
elevation: 0,
behavior: SnackBarBehavior.floating,
backgroundColor: Colors.transparent,
content: AwesomeSnackbarContent(
title: 'Oh Snap!',
message: "THis is an example error message that be shown in the body of snackbar!",
contentType: ContentType.failure,
)
);
ScaffoldMessenger.of(context)
..hideCurrentSnackBar()
..showSnackBar(snackBar);
},
child: const Text("Show Awesome SnackBar")
),
const SizedBox(height: 10,),
ElevatedButton(
onPressed: (){
final materialBanner = MaterialBanner(
elevation: 0,
backgroundColor: Colors.transparent,
forceActionsBelow: true,
content: AwesomeSnackbarContent(
title: 'Oh Hey!!',
message: "This is an example success message that will be shown in the body of materialBanner",
contentType: ContentType.success,
inMaterialBanner: true,
),
actions: const [SizedBox.shrink()]
);
ScaffoldMessenger.of(context)
..hideCurrentMaterialBanner()
..showMaterialBanner(materialBanner);
},
child: const Text("Show Awesome Material Banner"))
],
),
)
);
}
}
Leave a Reply