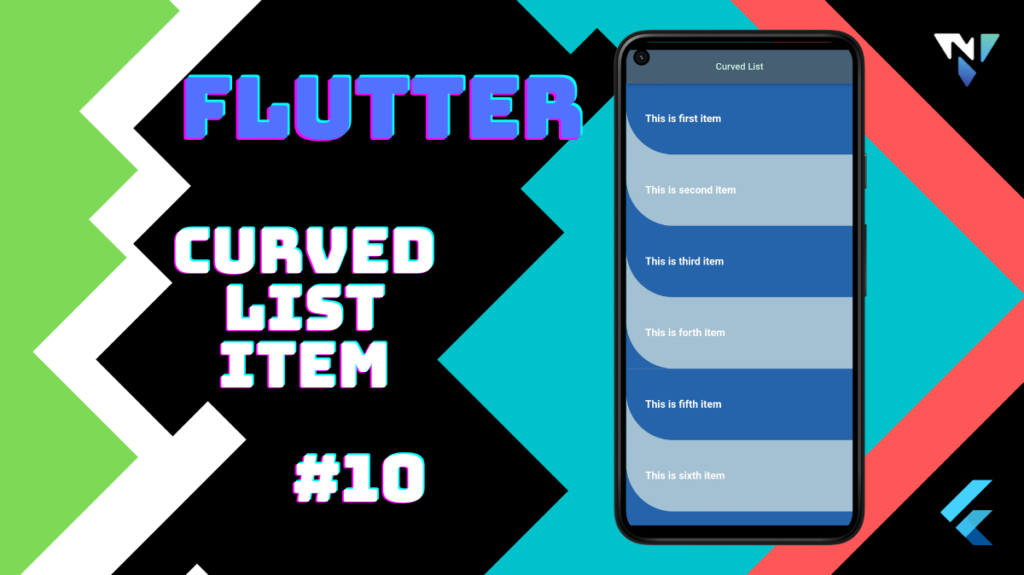
Tutorial and code of curved list item in flutter. Copy and paste the below code as per your requirements.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
MyApp() : super();
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: DefaultTabController(
length: 3,
child: Scaffold(
extendBodyBehindAppBar: true,
appBar: AppBar(
backgroundColor: const Color.fromRGBO(70, 95, 115 , 1),
title: const Text('Curved List',style: TextStyle(color: Color.fromRGBO(203,239,221,1)),),
centerTitle: true,
),
body:
SingleChildScrollView(
child: Stack(
children: [
ListView(
scrollDirection: Axis.vertical,
shrinkWrap: true,
physics: const ClampingScrollPhysics(),
children: const <Widget>[
CurvedListItem(
title: 'This is first item',
color: Color.fromRGBO(37,99,171,1),
nextColor: Color.fromRGBO(163,193,211,1),
),
CurvedListItem(
title: 'This is second item',
color: Color.fromRGBO(163,193,211,1),
nextColor: Color.fromRGBO(37,99,171,1),
),
CurvedListItem(
title: 'This is third item',
color: Color.fromRGBO(37,99,171,1),
nextColor: Color.fromRGBO(163,193,211,1),
),
CurvedListItem(
title: 'This is forth item',
color: Color.fromRGBO(163,193,211,1),
nextColor: Color.fromRGBO(37,99,171,1),
),
CurvedListItem(
title: 'This is fifth item',
color: Color.fromRGBO(37,99,171,1),
nextColor: Color.fromRGBO(163,193,211,1),
),
CurvedListItem(
title: 'This is sixth item',
color: Color.fromRGBO(163,193,211,1),
nextColor: Color.fromRGBO(37,99,171,1),
),
CurvedListItem(
title: 'This is seventh item',
color: Color.fromRGBO(37,99,171,1),
nextColor: Color.fromRGBO(163,193,211,1),
),
],
),
],
),
)
),
),
);
}
}
class CurvedListItem extends StatelessWidget {
const CurvedListItem({
required this.title,
required this.color,
required this.nextColor,
});
final String title;
final Color color;
final Color nextColor;
@override
Widget build(BuildContext context) {
return Container(
color: nextColor,
child: Container(
decoration: BoxDecoration(
color: color,
borderRadius: const BorderRadius.only(
bottomLeft: Radius.circular(80.0),
),
),
padding: const EdgeInsets.only(
left: 32,
top: 50.0,
bottom: 50,
),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Text(
title,
style: const TextStyle(
color: Colors.white,
fontSize: 22,
fontWeight: FontWeight.bold),
),
Row(),
]),
),
);
}
}
Leave a Reply