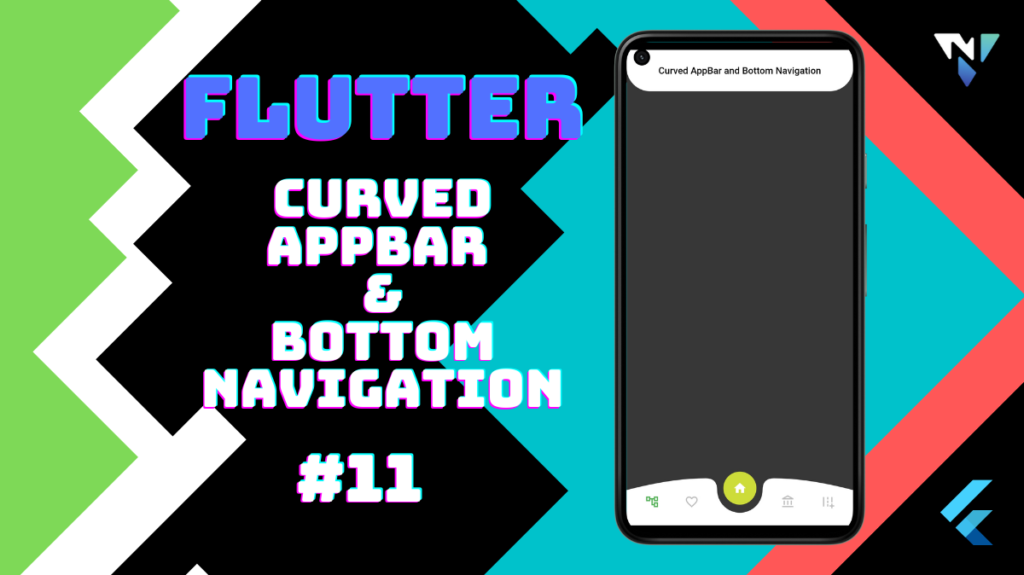
Tutorial and code of curved appbar & bottom navigation in flutter. Copy and paste the below code as per your requirements.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Curved Navbar',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: const BottomNavBar(),
);
}
}
class BottomNavBar extends StatefulWidget {
const BottomNavBar({Key? key}) : super(key: key);
@override
_BottomNavBarState createState() => _BottomNavBarState();
}
class _BottomNavBarState extends State<BottomNavBar> {
int currentIndex = 0;
setBottomBarIndex(index) {
setState(() {
currentIndex = index;
});
}
@override
Widget build(BuildContext context) {
final Size size = MediaQuery.of(context).size;
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.transparent,
elevation: 0.0,
toolbarHeight: 70,
title: const Text("Curved AppBar and Bottom Navigation",style: TextStyle(color: Colors.black),),
centerTitle: true,
flexibleSpace: Container(
decoration: const BoxDecoration(
borderRadius: BorderRadius.only(bottomLeft: Radius.circular(40),bottomRight: Radius.circular(40)),
color: Colors.white
),
),
),
backgroundColor: Colors.white.withAlpha(55),
body: Stack(
children: [
Positioned(
bottom: 0,
left: 0,
child: SizedBox(
width: size.width,
height: 80,
child: Stack(
children: [
CustomPaint(
size: Size(size.width, 80),
painter: BNBCustomPainter(),
),
Center(
heightFactor: 0.6,
child: FloatingActionButton(backgroundColor: Colors.lime, child: const Icon(Icons.home), elevation: 0.1, onPressed: () {}),
),
SizedBox(
width: size.width,
height: 80,
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
IconButton(
icon: Icon(
Icons.account_tree_outlined,
color: currentIndex == 0 ? Colors.green : Colors.grey.shade400,
),
onPressed: () {
setBottomBarIndex(0);
},
splashColor: Colors.white,
),
IconButton(
icon: Icon(
Icons.favorite_outline,
color: currentIndex == 1 ? Colors.green : Colors.grey.shade400,
),
onPressed: () {
setBottomBarIndex(1);
}),
Container(
width: size.width * 0.20,
),
IconButton(
icon: Icon(
Icons.account_balance_outlined,
color: currentIndex == 2 ? Colors.green : Colors.grey.shade400,
),
onPressed: () {
setBottomBarIndex(2);
}),
IconButton(
icon: Icon(
Icons.add_road_outlined,
color: currentIndex == 3 ? Colors.green : Colors.grey.shade400,
),
onPressed: () {
setBottomBarIndex(3);
}),
],
),
)
],
),
),
)
],
),
);
}
}
class BNBCustomPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
Paint paint = Paint()
..color = Colors.white
..style = PaintingStyle.fill;
Path path = Path();
path.moveTo(0, 20); // Start
path.quadraticBezierTo(size.width * 0.20, 0, size.width * 0.35, 0);
path.quadraticBezierTo(size.width * 0.40, 0, size.width * 0.40, 20);
path.arcToPoint(Offset(size.width * 0.60, 20), radius: const Radius.circular(20.0), clockwise: false);
path.quadraticBezierTo(size.width * 0.60, 0, size.width * 0.65, 0);
path.quadraticBezierTo(size.width * 0.80, 0, size.width, 20);
path.lineTo(size.width, size.height);
path.lineTo(0, size.height);
path.lineTo(0, 20);
canvas.drawShadow(path, Colors.black, 5, true);
canvas.drawPath(path, paint);
}
@override
bool shouldRepaint(CustomPainter oldDelegate) {
return false;
}
}
Leave a Reply