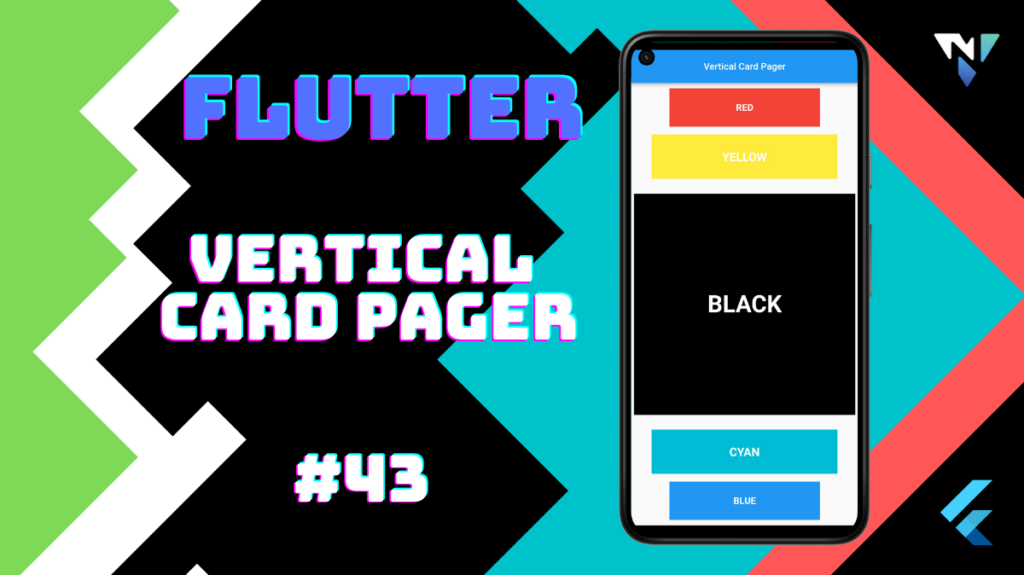
Tutorial and code of Vertical Card Pager in Flutter. Copy and paste the below code as per your requirements.
vertical_card_pager: ^1.5.0
import 'package:flutter/material.dart';
import 'package:vertical_card_pager/vertical_card_pager.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Vertical Card Pager',
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
final List<String> titles = [
"RED",
"YELLOW",
"BLACK",
"CYAN",
"BLUE",
"GREY",
];
final List<Widget> images = [
Container(
color: Colors.red,
),
Container(
color: Colors.yellow,
),
Container(
color: Colors.black,
),
Container(
color: Colors.cyan,
),
Container(
color: Colors.blue,
),
Container(
color: Colors.grey,
),
];
return Scaffold(
appBar: AppBar(
title: const Center(child: Text("Vertical Card Pager"),),
),
body: SafeArea(
child: Column(
children: <Widget>[
Expanded(
child: Container(
child: VerticalCardPager(
textStyle: const TextStyle(
color: Colors.white, fontWeight: FontWeight.bold),
titles: titles,
images: images,
onPageChanged: (page) {},
align: ALIGN.CENTER,
onSelectedItem: (index) {},
),
),
),
],
),
),
);
}
}
Leave a Reply