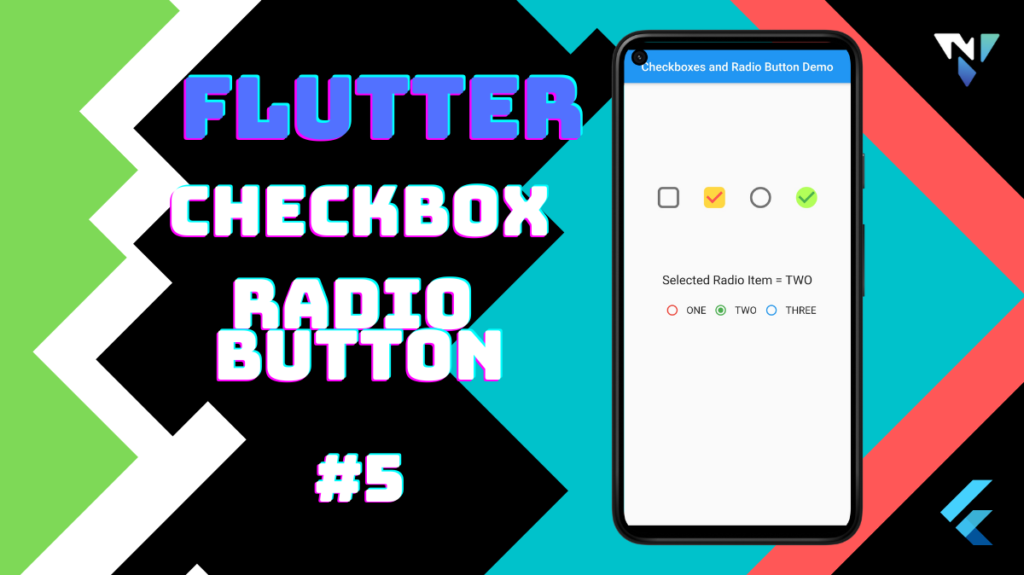
Tutorial and code of Checkbox and Radio Buttons in Flutter. Copy and paste the below code as per your requirements.
import 'package:flutter/material.dart';
void main() => runApp(MaterialApp(
debugShowCheckedModeBanner: false,
home: MyHomePage(),
));
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
bool a = false;
bool b = false;
bool c = false;
bool d = false;
bool e = false;
String radioButtonItem = 'ONE';
int id = 1;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Checkboxes and Radio Button Demo"),
centerTitle: true,
),
body: Center(
child: Column(
children: [
SizedBox(
height: 170,
),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Transform.scale(
scale: 2.0,
child: Checkbox(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(4.0),
),
activeColor: Colors.amberAccent,
checkColor: Colors.redAccent,
splashRadius: 20,
value: a,
onChanged: (bool value) {
setState(() {
a = value;
});
},
),
)
],
),
SizedBox(
width: 30,
),
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Transform.scale(
scale: 2.0,
child: Checkbox(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(4.0),
),
activeColor: Colors.amberAccent,
checkColor: Colors.redAccent,
splashRadius: 20,
value: b,
onChanged: (bool value) {
setState(() {
b = value;
});
},
),
)
],
),
SizedBox(
width: 30,
),
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Transform.scale(
scale: 2.0,
child: Checkbox(
shape: CircleBorder(),
activeColor: Colors.lightGreenAccent,
checkColor: Colors.green,
splashRadius: 20,
value: c,
onChanged: (bool value) {
setState(() {
c = value;
});
},
),
)
],
),
SizedBox(
width: 30,
),
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Transform.scale(
scale: 2.0,
child: Checkbox(
shape: CircleBorder(),
activeColor: Colors.lightGreenAccent,
checkColor: Colors.green,
splashRadius: 20,
value: d,
onChanged: (bool value) {
setState(() {
d = value;
});
},
),
)
],
),
],
),
SizedBox(
height: 90,
),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Column(
children: <Widget>[
Padding(
padding: EdgeInsets.all(14.0),
child: Text(
'Selected Radio Item = ' + '$radioButtonItem',
style: TextStyle(fontSize: 21))),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Radio(
fillColor: MaterialStateColor.resolveWith((states) => Colors.red),
value: 1,
groupValue: id,
onChanged: (val) {
setState(() {
radioButtonItem = 'ONE';
id = 1;
});
},
),
Text(
'ONE',
style: new TextStyle(fontSize: 17.0),
),
Radio(
fillColor: MaterialStateColor.resolveWith((states) => Colors.green),
value: 2,
groupValue: id,
onChanged: (val) {
setState(() {
radioButtonItem = 'TWO';
id = 2;
});
},
),
Text(
'TWO',
style: new TextStyle(
fontSize: 17.0,
),
),
Radio(
fillColor: MaterialStateColor.resolveWith((states) => Colors.blue),
value: 3,
groupValue: id,
onChanged: (val) {
setState(() {
radioButtonItem = 'THREE';
id = 3;
});
},
),
Text(
'THREE',
style: new TextStyle(fontSize: 17.0),
),
],
),
],
),
],
),
],
)));
}
}
Leave a Reply