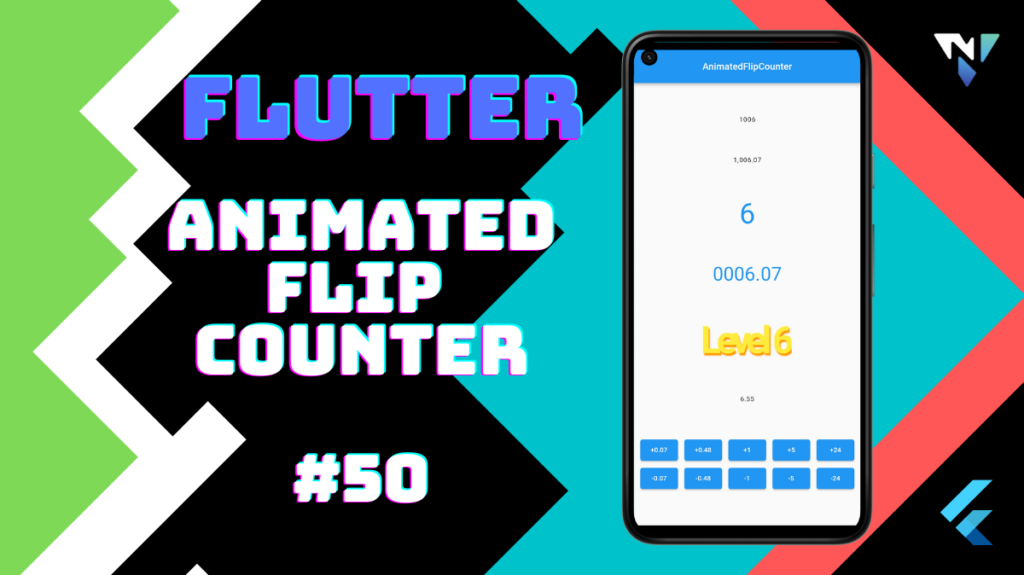
Tutorial and code of AnimatedFlipCounter in Flutter. Copy and paste the below code as per your requirements.
animated_flip_counter: ^0.2.4
import 'package:animated_flip_counter/animated_flip_counter.dart';
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
double _value = 0.0;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Center(child: Text("AnimatedFlipCounter"),),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
AnimatedFlipCounter(
value: 1000 + _value,
),
AnimatedFlipCounter(
value: 1000 + _value,
wholeDigits: 4,
fractionDigits: 2,
thousandSeparator: ',',
),
AnimatedFlipCounter(
value: _value,
duration: const Duration(seconds: 1),
curve: Curves.elasticOut,
textStyle: const TextStyle(fontSize: 60, color: Colors.blue),
),
AnimatedFlipCounter(
value: _value,
duration: const Duration(seconds: 1),
curve: Curves.bounceOut,
wholeDigits: 4,
fractionDigits: 2,
textStyle: const TextStyle(fontSize: 40, color: Colors.blue),
),
AnimatedFlipCounter(
value: _value,
prefix: "Level ",
textStyle: const TextStyle(
fontSize: 80,
fontWeight: FontWeight.bold,
letterSpacing: -8.0,
color: Colors.yellow,
shadows: [
BoxShadow(
color: Colors.orange,
offset: Offset(2, 4),
blurRadius: 4,
),
],
),
),
AnimatedFlipCounter(
value: _value + 0.48,
fractionDigits: 2,
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [0.07, 0.48, 1, 5, 24].map(_buildButton).toList(),
),
],
),
),
);
}
Widget _buildButton(num value) {
return Column(
children: [
ElevatedButton(
child: Text('+$value'),
onPressed: () => setState(() => _value += value),
),
ElevatedButton(
child: Text('-$value'),
onPressed: () => setState(() => _value -= value),
),
],
);
}
}