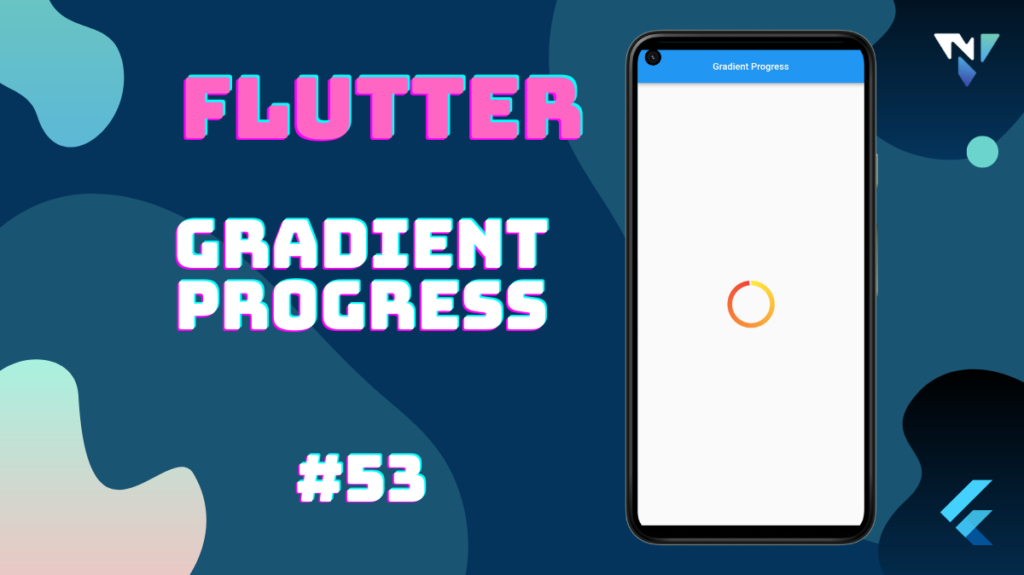
Tutorial and code of Gradient Progress in Flutter. Copy and paste the below code as per your requirements.
gradient_progress: ^0.1.0
import 'package:flutter/material.dart';
import 'package:gradient_progress/gradient_progress.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const ExampleHomePage(),
);
}
}
class ExampleHomePage extends StatefulWidget {
const ExampleHomePage({Key? key}) : super(key: key);
@override
_ExampleHomePageState createState() => _ExampleHomePageState();
}
class _ExampleHomePageState extends State<ExampleHomePage> with TickerProviderStateMixin{
late AnimationController _animationController;
@override
void initState() {
_animationController =
AnimationController(vsync: this, duration: const Duration(seconds: 3));
_animationController.addListener(() => setState(() {}));
_animationController.repeat();
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Center(child: Text("Gradient Progress"),),
),
body: Center(
child: GradientCircularProgressIndicator(
gradientColors: const [Colors.yellow, Colors.red],
radius: 40,
strokeWidth: 8.0,
value: Tween(begin: 0.0, end: 1.0)
.animate(CurvedAnimation(
parent: _animationController, curve: Curves.decelerate))
.value,
),
),
);
}
}