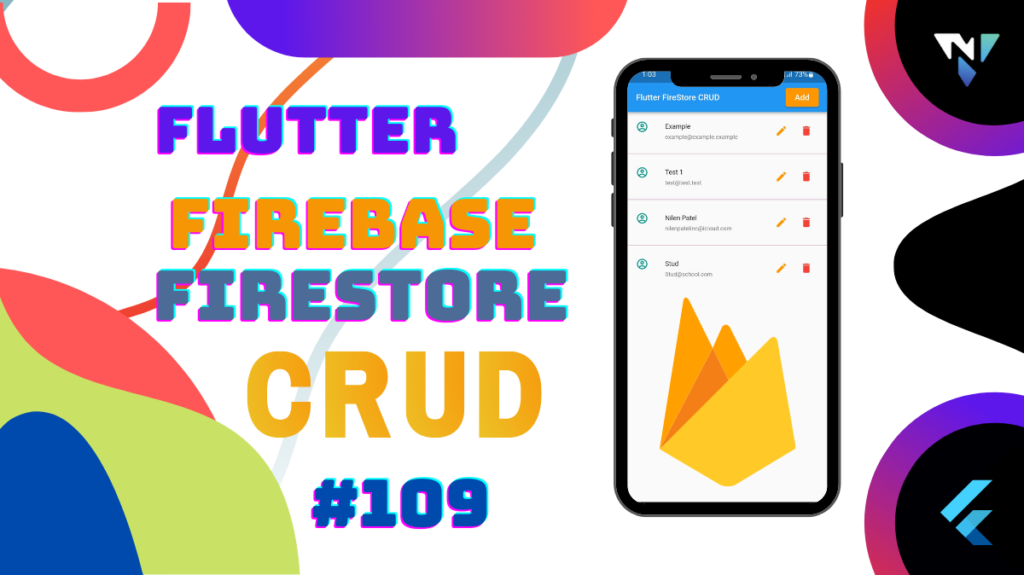
Flutter Firebase Cloud Firestore Complete Database CRUD | Beginner Tutorial. Copy and paste the below code as per your requirements.
main.dart
import 'package:example/pages/home_page.dart';
import 'package:firebase_core/firebase_core.dart';
import 'package:flutter/material.dart';
void main(){
WidgetsFlutterBinding.ensureInitialized();
runApp(MyApp());
}
class MyApp extends StatelessWidget{
final Future<FirebaseApp> _initialization = Firebase.initializeApp();
@override
Widget build(BuildContext context) {
return FutureBuilder(
future: _initialization,
builder: (context,snapshot){
if(snapshot.hasError)
{
print("Something Went Wrong.");
}
if(snapshot.connectionState == ConnectionState.done)
{
return MaterialApp(
title: 'Flutter Firestore CRUD',
theme: ThemeData(
primarySwatch: Colors.blue
),
debugShowCheckedModeBanner: false,
home: HomePage(),
);
}
return CircularProgressIndicator();
}
);
}
}
pages/home_page.dart
import 'package:example/pages/home_page.dart';
import 'package:firebase_core/firebase_core.dart';
import 'package:flutter/material.dart';
void main(){
WidgetsFlutterBinding.ensureInitialized();
runApp(MyApp());
}
class MyApp extends StatelessWidget{
final Future<FirebaseApp> _initialization = Firebase.initializeApp();
@override
Widget build(BuildContext context) {
return FutureBuilder(
future: _initialization,
builder: (context,snapshot){
if(snapshot.hasError)
{
print("Something Went Wrong.");
}
if(snapshot.connectionState == ConnectionState.done)
{
return MaterialApp(
title: 'Flutter Firestore CRUD',
theme: ThemeData(
primarySwatch: Colors.blue
),
debugShowCheckedModeBanner: false,
home: HomePage(),
);
}
return CircularProgressIndicator();
}
);
}
}
pages/list_student_page.dart
import 'package:cloud_firestore/cloud_firestore.dart';
import 'package:example/pages/update_student_page.dart';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class ListStudentPage extends StatefulWidget {
const ListStudentPage({Key? key}) : super(key: key);
@override
_ListStudentPageState createState() => _ListStudentPageState();
}
class _ListStudentPageState extends State<ListStudentPage> {
final Stream<QuerySnapshot> studentsStream =
FirebaseFirestore.instance.collection('students').snapshots();
CollectionReference students =
FirebaseFirestore.instance.collection('students');
Future<void> deleteUser(id) {
return students
.doc(id)
.delete()
.then((value) => print("User Deleted"))
.catchError((error) => print("Failed to Delete user: $error"));
}
@override
Widget build(BuildContext context) {
return StreamBuilder<QuerySnapshot>(
stream: studentsStream,
builder: (BuildContext context, AsyncSnapshot<QuerySnapshot> snapshot) {
if (snapshot.hasError) {
print("Something went wrong");
}
if (snapshot.connectionState == ConnectionState.waiting) {
return const Center(
child: CircularProgressIndicator(),
);
}
final List storedocs = [];
snapshot.data!.docs.map((DocumentSnapshot document)
{
Map a = document.data() as Map<String,dynamic>;
storedocs.add(a);
a['id'] = document.id;
}).toList();
return ListView.separated(
separatorBuilder: (context, index) => const Divider(color:Colors.pinkAccent),
itemCount: storedocs.length,
itemBuilder: (BuildContext context, int index){
return ListTile(
leading: const Icon(Icons.account_circle_outlined,color: Colors.teal,),
title: Text(storedocs[index]['name'].toString()),
subtitle: Text(storedocs[index]['email'].toString()),
trailing: Wrap(
children: [
IconButton(
onPressed: ()=>{
Navigator.push(context, MaterialPageRoute(builder: (context)=>UpdateStudentPage(id:storedocs[index]['id']))),
},
icon: const Icon(Icons.edit,color:Colors.orange),
),
IconButton(onPressed: ()=>{deleteUser(storedocs[index]['id'])},
icon: const Icon(Icons.delete,color:Colors.red))
],
),
);
}
);
});
}
}
pages/add_student_page.dart
import 'package:cloud_firestore/cloud_firestore.dart';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class AddStudentPage extends StatefulWidget{
const AddStudentPage({Key? key}):super(key:key);
@override
_AddStudentPageState createState() => _AddStudentPageState();
}
class _AddStudentPageState extends State<AddStudentPage>{
final _formKey = GlobalKey<FormState>();
var name = "";
var email = "";
var password = "";
final nameController = TextEditingController();
final emailController = TextEditingController();
final passwordController = TextEditingController();
@override
void dispose(){
nameController.dispose();
emailController.dispose();
passwordController.dispose();
super.dispose();
}
clearText(){
nameController.clear();
emailController.clear();
passwordController.clear();
}
CollectionReference students = FirebaseFirestore.instance.collection("students");
Future<void> addUser(){
return students.add({'name':name,'email':email,'password':password})
.then((value) => print('User Added'))
.catchError((error)=>print("Failed to Add user:$error"));
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text("Add New Student"),
),
body: Form(
key: _formKey,
child: Padding(
padding: const EdgeInsets.symmetric(vertical: 20,horizontal: 30),
child: ListView(
children: [
Container(
margin: const EdgeInsets.symmetric(vertical: 10),
child: TextFormField(
autofocus: false,
decoration: const InputDecoration(
labelText: 'Name: ',
labelStyle: TextStyle(fontSize: 20),
border: OutlineInputBorder(),
errorStyle: TextStyle(color: Colors.redAccent),
),
controller: nameController,
validator: (value){
if(value==null||value.isEmpty){
return 'Please Enter Name';
}
return null;
},
),
),
Container(
margin: const EdgeInsets.symmetric(vertical: 10),
child: TextFormField(
autofocus: false,
decoration: const InputDecoration(
labelText: 'Email: ',
labelStyle: TextStyle(fontSize: 20),
border: OutlineInputBorder(),
errorStyle: TextStyle(color: Colors.redAccent),
),
controller: emailController,
validator: (value){
if(value==null||value.isEmpty){
return 'Please Enter Email';
}
else if(!value.contains('@'))
{
return 'Please Enter Valid Email';
}
return null;
},
),
),
Container(
margin: const EdgeInsets.symmetric(vertical: 10),
child: TextFormField(
autofocus: false,
obscureText: true,
decoration: const InputDecoration(
labelText: 'Password: ',
labelStyle: TextStyle(fontSize: 20),
border: OutlineInputBorder(),
errorStyle: TextStyle(color: Colors.redAccent),
),
controller: passwordController,
validator: (value){
if(value==null||value.isEmpty){
return 'Please Enter Password';
}
return null;
},
),
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
ElevatedButton(
onPressed: (){
if(_formKey.currentState!.validate()){
setState(() {
name = nameController.text;
email = emailController.text;
password = passwordController.text;
addUser();
clearText();
});
}
},
child: const Text("Register", style: TextStyle(fontSize: 18.0),),
),
ElevatedButton(
onPressed: ()=>{clearText()},
child: const Text("Reset",style: TextStyle(fontSize: 18.0)),
style: ElevatedButton.styleFrom(primary: Colors.blueGrey),
)
],
)
],
),
),
),
);
}
}
pages/update_student_page.dart
import 'package:cloud_firestore/cloud_firestore.dart';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
class UpdateStudentPage extends StatefulWidget{
final String id;
const UpdateStudentPage({Key? key,required this.id}):super(key: key);
@override
_UpdateStudentPageState createState() => _UpdateStudentPageState();
}
class _UpdateStudentPageState extends State<UpdateStudentPage>{
final _formKey = GlobalKey<FormState>();
CollectionReference students = FirebaseFirestore.instance.collection('students');
Future<void> updateUser(id,name,email,password){
return students
.doc(id)
.update({'name':name,'email':email,'password':password})
.then((value) => print("User Updated"))
.catchError((error)=>print("Failed to update user:$error"));
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text("Update Student"),
),
body: Form(
key: _formKey,
child: FutureBuilder<DocumentSnapshot<Map<String,dynamic>>>(
future: FirebaseFirestore.instance
.collection('students')
.doc(widget.id)
.get(),
builder: (_,snapshot){
if(snapshot.connectionState==ConnectionState.waiting)
{
return const Center(
child: CircularProgressIndicator(),
);
}
var data = snapshot.data!.data();
var name = data!['name'];
var email = data!['email'];
var password = data!['password'];
return Padding(
padding: const EdgeInsets.symmetric(vertical: 20,horizontal: 30),
child: ListView(
children: [
Container(
margin: const EdgeInsets.symmetric(vertical: 10.0),
child: TextFormField(
initialValue: name,
autofocus: false,
onChanged: (value)=>name = value,
decoration: const InputDecoration(
labelText: 'Name: ',
labelStyle: TextStyle(fontSize: 20.0),
border: OutlineInputBorder(),
errorStyle: TextStyle(color: Colors.redAccent,fontSize: 15),
),
validator: (value){
if(value==null||value.isEmpty){
return 'Please Enter Name';
}
return null;
},
),
),
Container(
margin: const EdgeInsets.symmetric(vertical: 10),
child: TextFormField(
autofocus: false,
decoration: const InputDecoration(
labelText: 'Email: ',
labelStyle: TextStyle(fontSize: 20),
border: OutlineInputBorder(),
errorStyle: TextStyle(color: Colors.redAccent),
),
initialValue: email,
validator: (value){
if(value==null||value.isEmpty){
return 'Please Enter Email';
}
else if(!value.contains('@'))
{
return 'Please Enter Valid Email';
}
return null;
},
),
),
Container(
margin: const EdgeInsets.symmetric(vertical: 10),
child: TextFormField(
autofocus: false,
obscureText: true,
decoration: const InputDecoration(
labelText: 'Password: ',
labelStyle: TextStyle(fontSize: 20),
border: OutlineInputBorder(),
errorStyle: TextStyle(color: Colors.redAccent),
),
initialValue: password,
validator: (value){
if(value==null||value.isEmpty){
return 'Please Enter Password';
}
return null;
},
),
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
ElevatedButton(
onPressed: (){
if(_formKey.currentState!.validate())
{
updateUser(widget.id, name, email, password);
Navigator.pop(context);
}
},
child: const Text(
'Update',
style: TextStyle(fontSize: 18.0),
)),
ElevatedButton(
onPressed: ()=>{},
child: const Text('Reset',style: TextStyle(fontSize: 18.0),),
style: ElevatedButton.styleFrom(primary: Colors.blueGrey),
),
],
)
],
),
);
},
),
),
);
}
}