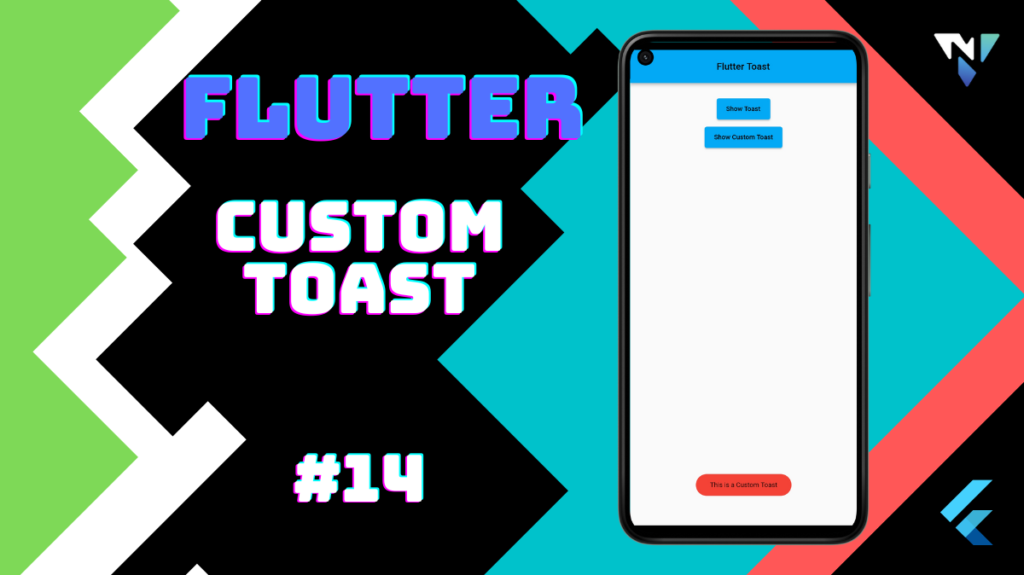
Tutorial and code of custom toast in flutter. Copy and paste the below code as per your requirements.
fluttertoast: ^8.0.7
import 'package:flutter/material.dart';
import 'package:fluttertoast/fluttertoast.dart';
void main()
{
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Toast',
theme: ThemeData(
primarySwatch: Colors.lightBlue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
_MyHomePageState createState()
{
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
late FToast fToast;
@override
void initState() {
super.initState();
fToast = FToast();
fToast.init(context);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Center(child: Text("Flutter Toast"),) ,
),
body: Container(
margin: const EdgeInsets.all(20),
alignment: Alignment.topCenter,
child: Column(
children: [
ElevatedButton(
child: const Text("Show Toast"),
onPressed: () {
showToast();
},
),
ElevatedButton(
child: const Text("Show Custom Toast"),
onPressed: () {
showCustomToast();
},
),
],
),
)
);
}
void showToast() {
Fluttertoast.showToast(
msg: "Hey There!!",
toastLength: Toast.LENGTH_SHORT,
);
}
showCustomToast() {
Widget toast = Container(
padding: const EdgeInsets.symmetric(horizontal: 24.0, vertical: 12.0),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(25.0),
color: Colors.red,
),
child: const Text("This is a Custom Toast"),
);
fToast.showToast(
child: toast,
toastDuration: const Duration(seconds: 3),
);
}
}