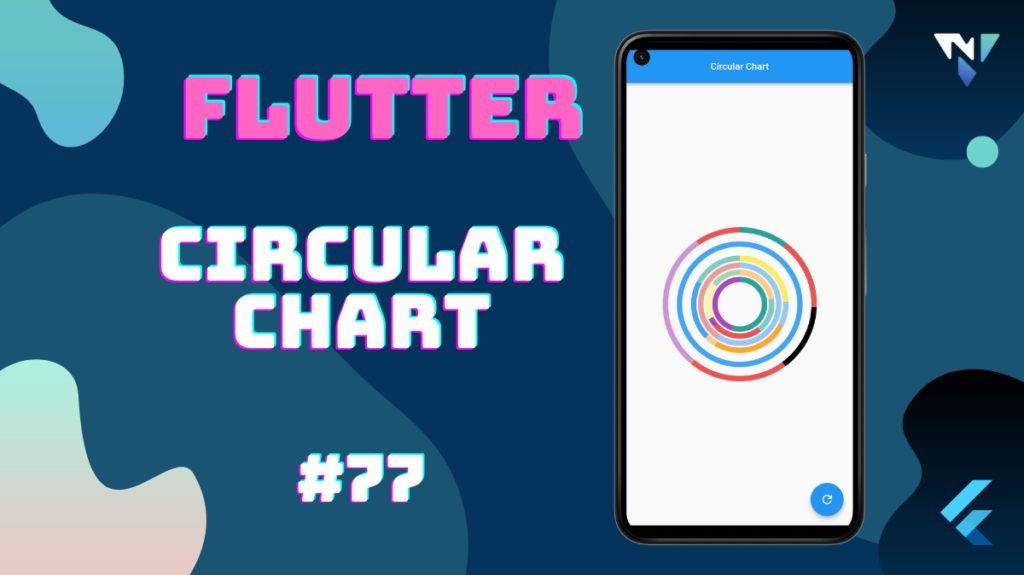
Tutorial and code of Circular Chart in Flutter. Copy and paste the below code as per your requirements.
awesome_circular_chart: ^1.0.0
import 'dart:math';
import 'package:awesome_circular_chart/awesome_circular_chart.dart';
import 'package:flutter/material.dart';
import 'dart:math' as Math;
void main() {
runApp(const MaterialApp(
debugShowCheckedModeBanner: false,
home: RandomizedRadialChartExample(),
));
}
class RandomizedRadialChartExample extends StatefulWidget {
const RandomizedRadialChartExample({Key? key}) : super(key: key);
@override
_RandomizedRadialChartExampleState createState() =>
_RandomizedRadialChartExampleState();
}
class _RandomizedRadialChartExampleState
extends State<RandomizedRadialChartExample> {
final GlobalKey<AnimatedCircularChartState> _chartKey =
GlobalKey<AnimatedCircularChartState>();
final _chartSize = const Size(300.0, 300.0);
final Math.Random random = Math.Random();
List<CircularStackEntry>? data;
@override
void initState() {
super.initState();
data = _generateRandomData();
}
double value = 50.0;
void _randomize() {
setState(() {
data = _generateRandomData();
_chartKey.currentState!.updateData(data!);
});
}
List<CircularStackEntry> _generateRandomData() {
int stackCount = random.nextInt(10);
List<CircularStackEntry> data = List.generate(stackCount, (i) {
int segCount = random.nextInt(10);
List<CircularSegmentEntry> segments = List.generate(segCount, (j) {
Color? randomColor = ColorPalette.primary.random(random);
return CircularSegmentEntry(random.nextDouble(), randomColor);
});
return CircularStackEntry(segments);
});
return data;
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Center(child: Text('Circular Chart'),),
),
body: Center(
child: AnimatedCircularChart(
key: _chartKey,
size: _chartSize,
initialChartData: data,
chartType: CircularChartType.Radial,
),
),
floatingActionButton: FloatingActionButton(
onPressed: _randomize,
child: const Icon(Icons.refresh),
),
);
}
}
class ColorPalette {
static final ColorPalette primary = ColorPalette(<Color?>[
Colors.blue[400],
Colors.blue[200],
Colors.red[400],
Colors.red[200],
Colors.green[400],
Colors.green[200],
Colors.yellow[400],
Colors.yellow[200],
Colors.purple[400],
Colors.purple[200],
Colors.orange[400],
Colors.orange[200],
Colors.teal[400],
Colors.teal[200],
Colors.black,
]);
ColorPalette(List<Color?> colors) : _colors = colors {
assert(colors.isNotEmpty);
}
final List<Color?> _colors;
Color? operator [](int index) => _colors[index % length];
int get length => _colors.length;
Color? random(Random random) => this[random.nextInt(length)];
}