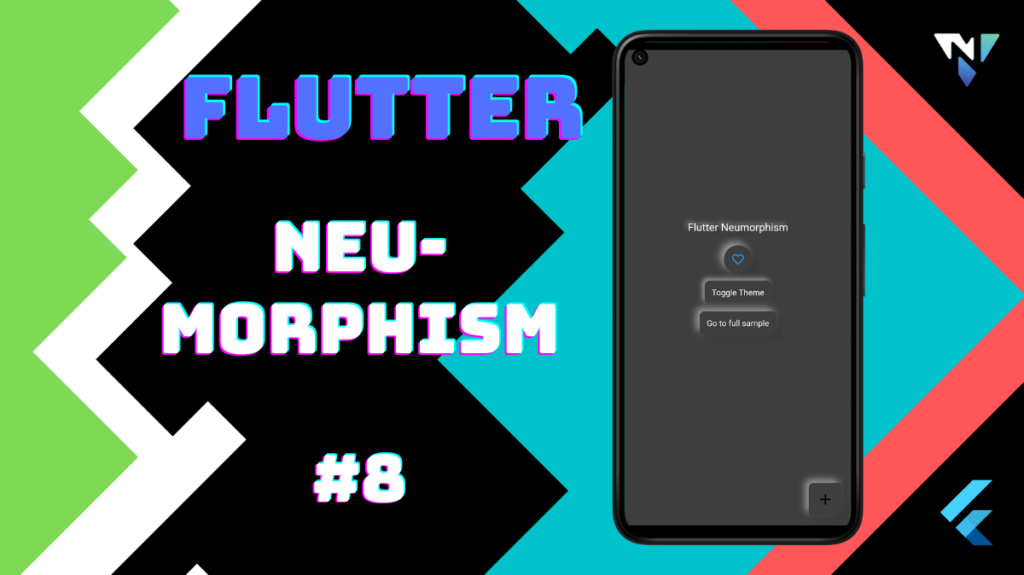
Tutorial and code of neumorphism in flutter. Copy and paste the below code as per your requirements.
pubspec.yaml
name: neumorphism
description: A new Flutter project.
publish_to:
version: 1.0.0+1
environment:
sdk: ">=2.15.1 <3.0.0"
dependencies:
flutter:
sdk: flutter
cupertino_icons: ^1.0.2
flutter_neumorphic: ^3.0.3
dev_dependencies:
flutter_test:
sdk: flutter
flutter_lints: ^1.0.0
flutter:
uses-material-design: true
main.dart
import 'package:flutter_neumorphic/flutter_neumorphic.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const NeumorphicApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Neumorphism',
themeMode: ThemeMode.light,
theme: NeumorphicThemeData(
baseColor: Color(0xFFFFFFFF),
lightSource: LightSource.topLeft,
depth: 10,
),
darkTheme:NeumorphicThemeData(
baseColor: Color(0xFF3E3E3E),
lightSource: LightSource.topLeft,
depth: 6,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
Widget build(BuildContext context) {
return Scaffold(
floatingActionButton: NeumorphicFloatingActionButton(
child: const Icon(Icons.add, size: 30),
onPressed: () {},
),
backgroundColor: NeumorphicTheme.baseColor(context),
body: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
NeumorphicText(
"Flutter Neumorphism",
style: const NeumorphicStyle(
depth: 4, //customize depth here
color: Colors.white,
),
textStyle: NeumorphicTextStyle(
fontSize: 18,
),
),
const SizedBox(
height: 20,
),
NeumorphicButton(
onPressed: () {
print("onClick");
},
style: const NeumorphicStyle(
shape: NeumorphicShape.flat,
boxShape: NeumorphicBoxShape.circle(),
),
padding: const EdgeInsets.all(12.0),
child: Icon(
Icons.favorite_border,
color: _iconsColor(context),
),
),
NeumorphicButton(
margin: EdgeInsets.only(top: 12),
onPressed: () {
NeumorphicTheme.of(context)?.themeMode =
NeumorphicTheme.isUsingDark(context)
? ThemeMode.light
: ThemeMode.dark;
},
style: NeumorphicStyle(
shape: NeumorphicShape.flat,
boxShape:
NeumorphicBoxShape.roundRect(BorderRadius.circular(8)),
),
padding: const EdgeInsets.all(12.0),
child: Text(
"Toggle Theme",
style: TextStyle(color: _textColor(context)),
)),
NeumorphicButton(
margin: const EdgeInsets.only(top: 12),
onPressed: () {
},
style: NeumorphicStyle(
shape: NeumorphicShape.flat,
boxShape:
NeumorphicBoxShape.roundRect(BorderRadius.circular(8)),
//border: NeumorphicBorder()
),
padding: const EdgeInsets.all(12.0),
child: Text(
"Go to full sample",
style: TextStyle(color: _textColor(context)),
)),
],
),
),
);
}
Color? _iconsColor(BuildContext context) {
final theme = NeumorphicTheme.of(context);
if (theme!.isUsingDark) {
return theme!.current!.accentColor;
} else {
return null;
}
}
Color _textColor(BuildContext context) {
if (NeumorphicTheme.isUsingDark(context)) {
return Colors.white;
} else {
return Colors.black;
}
}
}