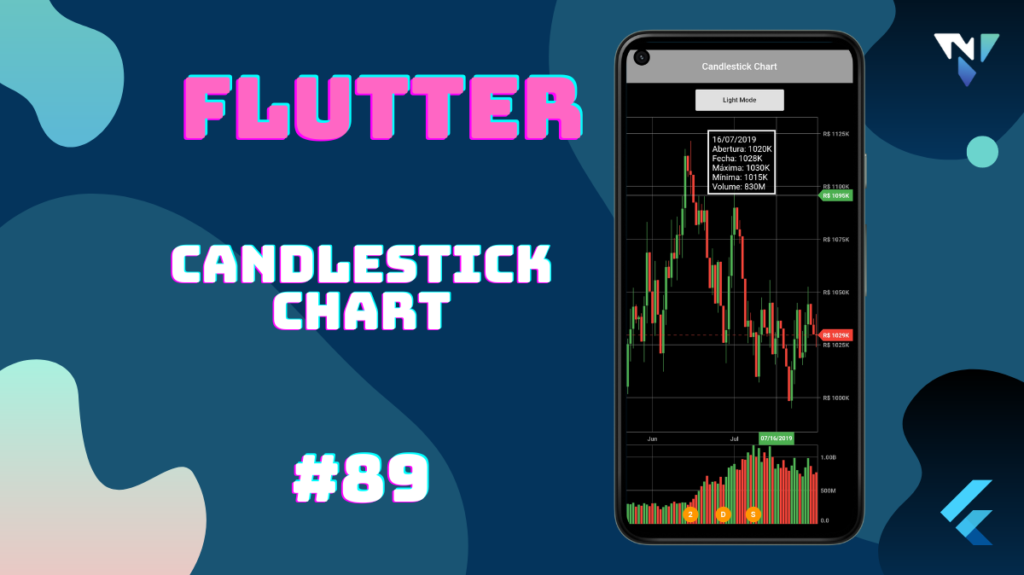
Tutorial and code of Awesome Slider in Flutter. Copy and paste the below code as per your requirements.
awesome_slider: ^0.1.0
import 'package:flutter/material.dart';
import 'package:awesome_slider/awesome_slider.dart';
void main() => runApp(const MainApp());
class MainApp extends StatefulWidget {
const MainApp({Key? key}) : super(key: key);
@override
_MainAppState createState() => _MainAppState();
}
class _MainAppState extends State<MainApp> {
double valueOnTextWidget = 0.0;
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
appBar: AppBar(title: const Center(child: Text("Awesome Slider"),),),
body: Container(
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [
Colors.red,
const Color(0xFF100887).withOpacity(0.93)
],
begin: Alignment.topLeft,
end: Alignment.bottomRight,
stops: const [0.0, 0.5],
),
),
child: Column(
children: <Widget>[
const SizedBox(
height: 250.0,
),
Text(
valueOnTextWidget.round().toString(),
style: const TextStyle(color: Colors.white, fontSize: 150.0),
),
const SizedBox(
height: 80.0,
),
AwesomeSlider(
value: valueOnTextWidget,
min: 0.0,
max: 100.0,
thumbColor: const Color(0xFF100887),
roundedRectangleThumbRadius: 25.0,
thumbSize: 100.0,
topLeftShadow: true,
topLeftShadowColor: Colors.lightBlueAccent,
topLeftShadowBlur: const MaskFilter.blur(BlurStyle.normal, 11.0),
bottomRightShadow: true,
bottomRightShadowColor: Colors.white.withOpacity(0.5),
bottomRightShadowBlur: const MaskFilter.blur(BlurStyle.normal, 11.0),
activeLineStroke: 2.0,
activeLineColor: Colors.blueAccent,
inactiveLineColor: Colors.white,
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: const <Widget>[
Icon(
Icons.arrow_back_ios,
color: Colors.white,
size: 28.0,
),
SizedBox(width: 10.0),
Icon(
Icons.arrow_forward_ios,
color: Colors.white,
size: 28.0,
)
],
),
onChanged: (double value) {
setState(() {
valueOnTextWidget = value;
});
},
),
],
),
),
),
);
}
}