Tutorial and code of Round Slider in Flutter. Copy and paste the below code as per your requirements.
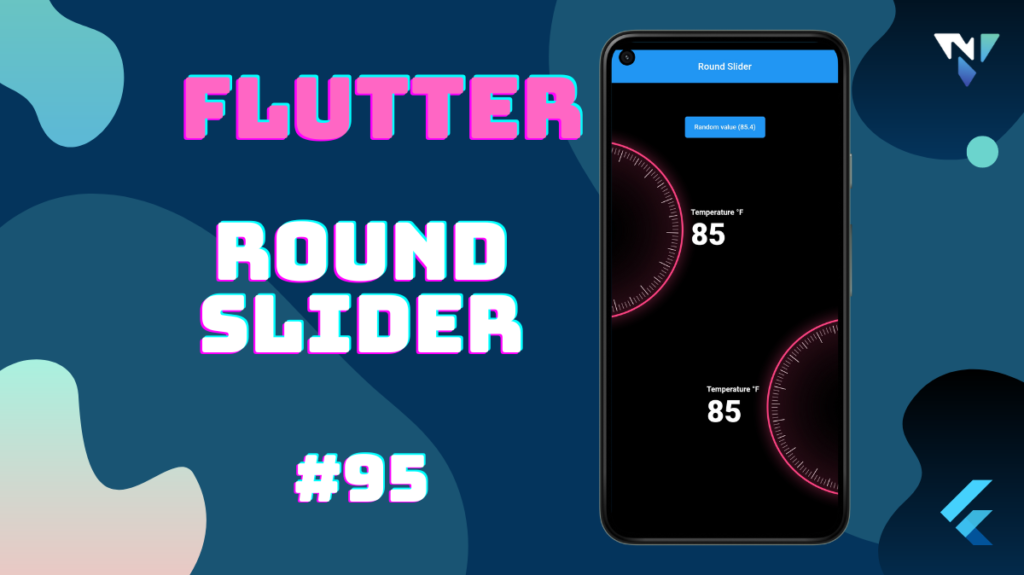
flutter_round_slider: ^1.0.0
import 'dart:math';
import 'package:flutter/material.dart';
import 'package:flutter_round_slider/flutter_round_slider.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
double value = 0;
final valueTween = Tween<double>(begin: -120, end: 120);
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.black,
appBar: AppBar(
title: const Center(child: Text('Round Slider'),),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ElevatedButton(
onPressed: () {
setState(() {
value = Random.secure().nextDouble();
});
},
child: Text(
'Random value (${valueTween.transform(value).toStringAsFixed(1)})')),
Row(
children: [
RoundSlider(
style: const RoundSliderStyle(
alignment: RoundSliderAlignment.left,
),
value: value,
onChanged: (double value) {
setState(() {
this.value = value;
});
},
),
Padding(
padding: const EdgeInsets.only(left: 14.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
const Text(
'Temperature °F',
style: TextStyle(
fontSize: 16,
color: Colors.white,
fontWeight: FontWeight.bold,
),
),
Text(
'${valueTween.transform(value).round()}',
style: const TextStyle(
fontSize: 64,
color: Colors.white,
fontWeight: FontWeight.w900,
),
),
],
),
),
],
),
Row(
mainAxisAlignment: MainAxisAlignment.end,
children: [
Padding(
padding: const EdgeInsets.only(right: 14.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
const Text(
'Temperature °F',
style: TextStyle(
fontSize: 16,
color: Colors.white,
fontWeight: FontWeight.bold,
),
),
Text(
'${valueTween.transform(value).round()}',
style: const TextStyle(
fontSize: 64,
color: Colors.white,
fontWeight: FontWeight.w900,
),
),
],
),
),
RoundSlider(
style: const RoundSliderStyle(
alignment: RoundSliderAlignment.right,
),
value: value,
onChanged: (double value) {
setState(() {
this.value = value;
});
},
),
],
),
],
),
);
}
}