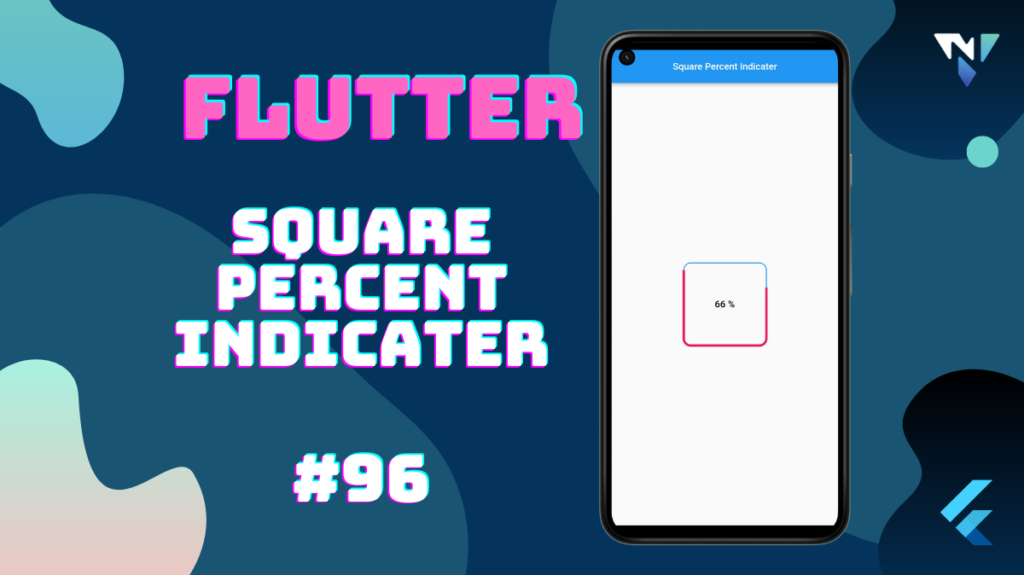
Tutorial and code of Square Percent Indicater in Flutter. Copy and paste the below code as per your requirements.
square_percent_indicater: ^0.1.0
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:square_percent_indicater/square_percent_indicater.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int value = 0;
late Timer timer;
@override
void initState() {
super.initState();
timer = Timer.periodic(const Duration(milliseconds: 20), (Timer t) {
setState(() {
value = (value + 1) % 100;
});
});
}
@override
void dispose() {
timer.cancel();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Center(child: Text("Square Percent Indicater"),),),
body: Center(
child: SquarePercentIndicator(
width: 140,
height: 140,
reverse: true,
borderRadius: 12,
shadowWidth: 1.5,
progressWidth: 4,
shadowColor: Colors.blue,
progressColor: Colors.pink,
progress: value / 100,
child: Center(
child: Text(
"$value %",
style: const TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
)),
),
),
);
}
}